mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2025-07-12 12:17:52 +00:00
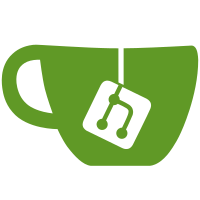
* Initial DrawTexture support & Advanced Present * TODO: Get Scissors Working * Chnage scissor state management * Rebase problems… * Rebase fixes again * Update DrawTexture + Fix Topology * Fix flipping * Add clear action support * Cleanup
31 lines
823 B
Metal
31 lines
823 B
Metal
#include <metal_stdlib>
|
|
|
|
using namespace metal;
|
|
|
|
struct CopyVertexOut {
|
|
float4 position [[position]];
|
|
float2 uv;
|
|
};
|
|
|
|
vertex CopyVertexOut vertexMain(uint vid [[vertex_id]],
|
|
const device float* texCoord [[buffer(0)]]) {
|
|
CopyVertexOut out;
|
|
|
|
int low = vid & 1;
|
|
int high = vid >> 1;
|
|
out.uv.x = texCoord[low];
|
|
out.uv.y = texCoord[2 + high];
|
|
out.position.x = (float(low) - 0.5f) * 2.0f;
|
|
out.position.y = (float(high) - 0.5f) * 2.0f;
|
|
out.position.z = 0.0f;
|
|
out.position.w = 1.0f;
|
|
|
|
return out;
|
|
}
|
|
|
|
fragment float4 fragmentMain(CopyVertexOut in [[stage_in]],
|
|
texture2d<float, access::sample> texture [[texture(0)]],
|
|
sampler sampler [[sampler(0)]]) {
|
|
return texture.sample(sampler, in.uv);
|
|
}
|